Progress Bar in Tk (tkinter)
A progress bar is useful to display the status of an operation or task. The ttk.Progressbar
widget can indicate the evolution of a certain process (for example, downloading a file from the Internet) or simply represent that an operation is being executed, in those cases in which the remaining time is unknown.
Standard Progress Bar¶
Let's start with a little code that creates a window with a horizontal progress bar.
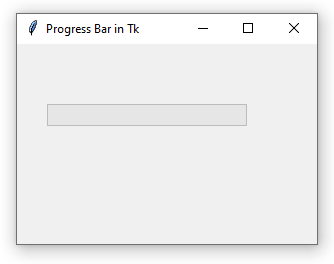
We can also indicate a vertical orientation via the orient
parameter:
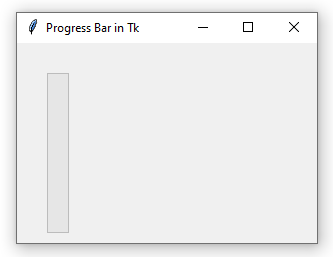
The default value for orient
is tk.HORIZONTAL
.
Note that, depending on the chosen orientation, the length of the widget is determined by the width
(horizontal) or height
(vertical) argument. To set the length regardless of its orientation, the length
parameter can be used.
Back to the first code, by default the progress bar is set to represent a percentage. That is, it takes values from 0 (totally empty) to 100 (totally full). We set the progress via the step
function by indicating a value between the aforementioned range.
Once this method is executed, the progress will be halfway:
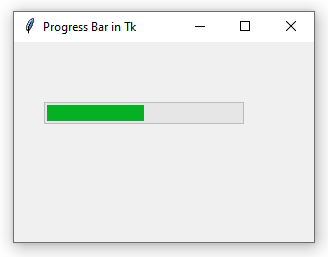
If the argument is omitted, the increment is equal to 1.0
(note that the function can take floating point numbers).
A peculiarity in Tk is that a progress bar cannot be shown "complete". When it reaches its maximum value, the progress returns to 0. For this reason, the following code shows an empty bar:
Therefore, to display the (almost) complete bar, it will be convenient to use a value close to the maximum (which by default is 100):
The maximum value of a progress bar can be set via the maximum
parameter:
In this case, since the maximum value is 200, a call to progressbar.step(50)
will set a quarter of the progress.
The value of a progress bar can also be handled by a variable, such as tk.IntVar
, by indicating it in the variable
parameter.
In this case, to set the progress of the progress bar, you must call the set()
method of the assigned variable:
Note that set()
sets the value of the progress bar, while step()
increases it. Two calls to step(10)
set the progress at 20, but two calls to set(10)
simply keep it at 10.
Indeterminate Progress Bar¶
An indeterminate progress bar is useful when we want to tell the user that something is happening, but we don't know exactly how long it will take. When using the indeterminate mode, the progress bar shows a small "load" scrolling back and forth.
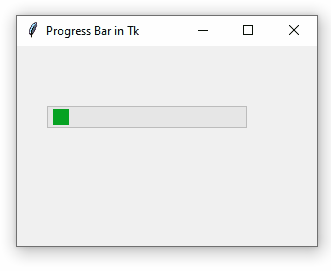
The mode
parameter defaults to "determinate"
.
We can alter the movement speed by indicating the milliseconds (50 by default):
To stop the movement, use the stop()
method:
Examples¶
Download a file via HTTP and display the progress:
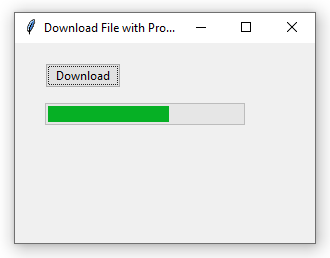
Note that this code example lacks thread safety. See Background Tasks with Tk (tkinter).