Checkbox (Checkbutton) in Tk (tkinter)
The ttk.Checkbutton
widget (available in Tk 8.5+), also known as Checkbox, is a type of button that allows two opposite states to be represented (checked/unchecked, on/off, yes/no, etc.) by the presence or absence of a check mark.
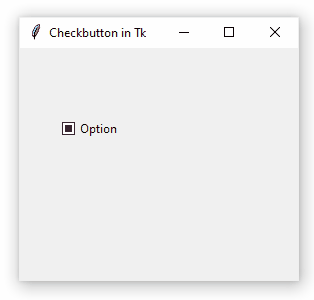
The following code creates a checkbox with the text "Option" as shown in the image above.
|
import tkinter as tk
|
|
from tkinter import ttk
|
|
|
|
root = tk.Tk()
|
|
root.title("Checkbutton in Tk")
|
|
checkbutton = ttk.Checkbutton(text="Option")
|
|
checkbutton.place(x=40, y=70)
|
|
root.mainloop()
|
Note, however, that the widget is initialized with an indeterminate state. In order to get access to the state of a checkbox it is first necessary to create a variable that can store its value. For convenience we use tk.BooleanVar
, which will represent the checked state as True
and unchecked as False
.
By default, tk.BooleanVar
is initialized with False
, so the widget is initially unchecked.
You can read and change a checkbutton state by using the variable's set()
and get()
methods, respectively. For example, the following code uses the command
parameter to print the value of the checkbutton each time the widget is clicked.
If you want to initialize the widget with a checked state, set the value of the variable before creating the checkbutton:
|
checkbutton_value = tk.BooleanVar()
|
|
checkbutton_value.set(True)
|
|
checkbutton = ttk.Checkbutton(text="Option", variable=checkbutton_value)
|
Or simply:
|
checkbutton_value = tk.BooleanVar(value=True)
|
|
checkbutton = ttk.Checkbutton(text="Option", variable=checkbutton_value)
|
You can simulate a state change in the widget by using the invoke()
method. A call to invoke()
is similar to the user clicking on the checkbox. For example, the following code creates a button widget that changes the state of the checkbutton when clicked.
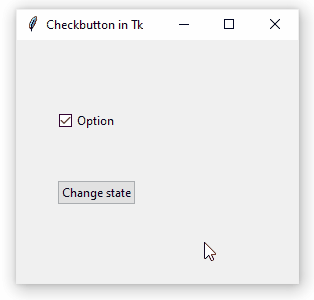
The difference between calling invoke()
and changing the value manually using checkbox_value.set()
is that the first method completely simulates a state change, which includes the checkbox_clicked
function call or that of any other registered event handler.
Wrapper Class¶
In most cases you'll probably want to use the following wrapper class to work with checkbuttons.
In our previous code, we had to create two objects: the widget itself (checkbutton
) and a variable that stores its state (checkbox_value
). This wrapper class handles the tk.BooleanVar
instance internally while exposing the checked()
method to query the checkbutton state, and check()
and uncheck()
to change it.
Custom State Values¶
A boolean variable is often used to represent the states of a checkbutton, thus checked equals to True
and unchecked to False
. However, you can choose any other values for those states. For example, a checkbutton instance could store its state as strings, namely "on"
and "off"
:
Thus, checkbutton_value.get()
will return one of the two values specified in the onvalue
and offvalue
parameters.
It is not possible to determine what value get()
will return if the user has not already clicked on the checkbox, but it is certain that it will be a value other than onvalue
and offvalue
. In our example, we can consider:
chosen
will be equal to False
when no choice has been made yet and True
otherwise.