By default tkinter
textboxes (ttk.Entry
and tk.Entry
classes) provide the functionality to copy, cut and paste content via keyboard shortcuts. However, users also expect the classic context menu, which is not shipped out of the box in Tk.
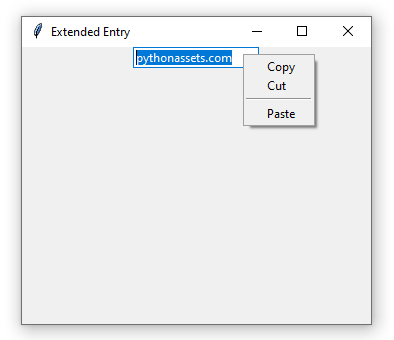
But manually implementing a context menu alike is a more or less simple task. In the following code we create a new class called EntryEx
that inherits all the functionality of ttk.Entry
, but also adds copy, cut and paste options using tk.Menu
.
|
import tkinter as tk
|
|
from tkinter import ttk
|
|
|
|
|
|
class EntryEx(ttk.Entry):
|
|
"""
|
|
Extended entry widget that includes a context menu
|
|
with Copy, Cut and Paste commands.
|
|
"""
|
|
|
|
def __init__(self, *args, **kwargs):
|
|
super().__init__(*args, **kwargs)
|
|
self.menu = tk.Menu(self, tearoff=False)
|
|
self.menu.add_command(label="Copy", command=self.popup_copy)
|
|
self.menu.add_command(label="Cut", command=self.popup_cut)
|
|
self.menu.add_separator()
|
|
self.menu.add_command(label="Paste", command=self.popup_paste)
|
|
self.bind("<Button-3>", self.display_popup)
|
|
|
|
def display_popup(self, event):
|
|
self.menu.post(event.x_root, event.y_root)
|
|
|
|
def popup_copy(self):
|
|
self.event_generate("<<Copy>>")
|
|
|
|
def popup_cut(self):
|
|
self.event_generate("<<Cut>>")
|
|
|
|
def popup_paste(self):
|
|
self.event_generate("<<Paste>>")
|
|
|
|
|
|
root = tk.Tk()
|
|
root.title("Extended Entry")
|
|
root.geometry("400x300")
|
|
entry = EntryEx()
|
|
entry.pack()
|
|
root.mainloop()
|
That's all! Just create your textboxes from our new EntryEx
class.